During WWDC 2021, Apple introduced the new APIs that make number formatting a lot easier. Before iOS 15, we could use the String(format:, _)
, but we had to remember the syntax of the format string:
let number: Float = 3.14159
let string = String(format: "%.2f", number)
// 3.14
From iOS 15 (and other operating systems unveiled during WWDC 2021), we can use the new formatted()
method:
let number: Float = 3.14159
let string = number.formatted()
// 3.14159 for en_US Locale
// 3,14159 for pl Locale
By default, it will return the string representation of the number that respects the current user's Locale. That' what Apple calls a Sensible Default.
Of course, we can customize the output. For example, we can set the length of the fraction part:
let number: Float = 3.14159
let string = number.formatted(.number.precision(.fractionLength(2)))
// 3.14
Using the .precision()
modifier we can also set the number of leading zeros:
let number: Float = 3.14159
let string = number.formatted(.number.precision(.integerLength(2)))
// 03.251299
If we need to customize both the integer and fraction parts, Apple has us covered:
let number: Float = 3.14159
let string = number.formatted(.number.precision(.integerAndFractionLength(integer: 2, fraction: 2)))
// 03.25
Formatter allows us to force a display of the decimal separator, even if the number does not have a fraction part:
let number: Float = 3
let string = number.formatted(.number.decimalSeparator(strategy: .always))
// 3.
In some locales, to increase readability, the digits are being grouped. We can customize this behavior with the .grouping
modifier:
let number: Float = 3900500.22
let grouped = number.formatted()
// 3,900,500.25
let notGrouped = number.formatted(.number.grouping(.never))
// 3900500.25
We can also customize the sign symbol:
let number: Float = 3.14159
let string = number.formatted(number.sign(strategy: .always()))
// +3.14159
And finally, we can format a number using a scientific notation:
let number: Float = 3.14159
let string = number.formatted(.number.notation(.scientific))
// 3.251299E0
The number formatters allow us to round a number, so we don't have to do this manually:
let number: Float = 3.14159
let roundedDown = number.formatted(.number.rounded(rule: .towardZero, increment: 0.1))
// 3.1
let roundedUp = number.formatted(.number.rounded(rule: .awayFromZero, increment: 0.1))
// 3.2
And, if for some reason, we need to scale the number, we can do this with .scale()
modifier:
let number: Float = 3.14159
let string = number.formatted(.scale(100))
// 314.159
How to format a number as percents
To format a number as a percentage, we can use the .percent
style:
let number = 25
let string = number.formatted(.percent)
// 25%
This style will automatically format the number and append the %
symbol. Of course, we can customize the number formatting using the modifiers mentioned above.
How to format a number as a currency
To format a number as a currency, we can use the .currency
style:
let number: Float = 3.14159
let currency = number.formatted(.currency(code: "USD"))
// $3.14 for en_US Locale
// 3,14 USD for pl Locale
The currency style respects the user's Locale, so for the en_US
Locale, we will get the $3.14
, and for the pl
Locale, we will have the 3,14 USD
, which is the currency format used in Poland.
That's it. I hope this cheat sheet was helpful. If you like the articles like this, maybe you would like to learn how to format a Date into a String:
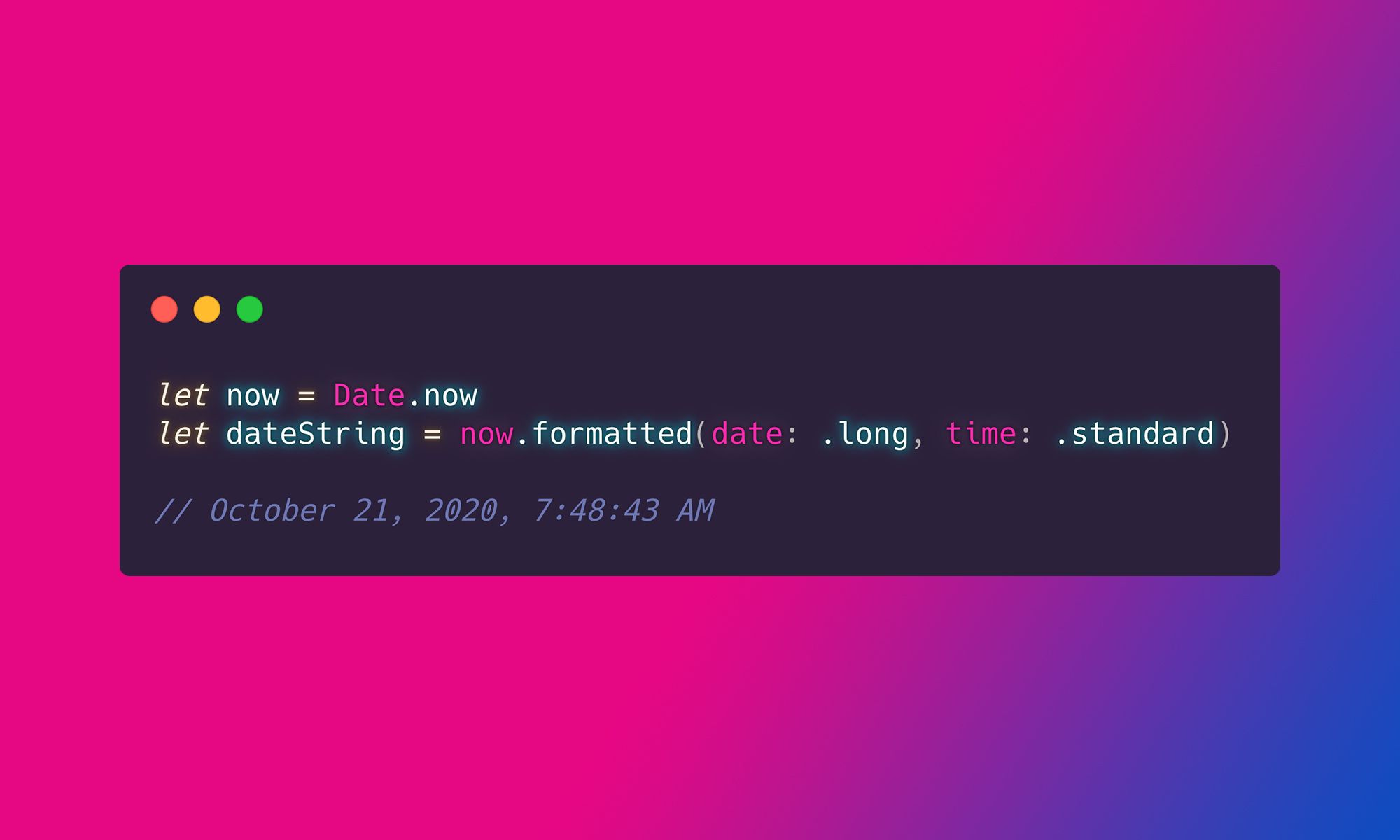
Comments
Anything interesting to share? Write a comment.