During WWDC 2021, Apple introduced the new APIs that make String to Date conversion a lot easier.
Before iOS 15, we had to create a DateFormatter
and later use it to convert a String into a Date:
let dateFormatter: DateFormatter = {
let formatter = DateFormatter()
formatter.timeZone = TimeZone.current // Europe/Warsaw (UTC+2)
formatter.dateFormat = "yyyy-MM-dd HH:mm:ss"
return formatter
}()
let dateString = "2020-10-21 18:42:56"
let date = dateFormatter.date(from: dateString)
// 2020-10-21 16:42:56 +0000 UTC
// 2020-10-21 18:42:56 (Warsaw, UTC+2)
From iOS 15, we can condense the above code to just three lines:
let dateString = "2020-10-21 18:42:56"
let strategy = Date.ISO8601FormatStyle().year().month().day().dateSeparator(.dash).dateTimeSeparator(.space).time(includingFractionalSeconds: false).timeSeparator(.colon)
let date = try? Date(dateString, strategy: strategy)
// 2020-10-21 16:42:56 +0000 UTC
// 2020-10-21 18:42:56 (Warsaw, UTC+2)
The Date
structure now has a special initializer that takes a string and a parsing strategy. In the case above, we customized the existing ISO8601FormatStyle
, but we can define our custom strategy:
let dateString = "2020-10-21 18:42:56"
let customStrategy = Date.ParseStrategy(
format: "\(year: .defaultDigits)-\(month: .twoDigits)-\(day: .twoDigits) \(hour: .twoDigits(clock: .twentyFourHour, hourCycle: .zeroBased)):\(minute: .twoDigits):\(second: .twoDigits)",
timeZone: .current
)
let date = try? Date(dateString, strategy: customStrategy)
// 2020-10-21 16:42:56 +0000 UTC
// 2020-10-21 18:42:56 (Warsaw, UTC+2)
As you can see, we have the full power of the DateFormatter
, but we don't have to remember the format syntax.
That's it. If you want to know how to convert and format a Date into a String, check out my other article:
How to format a Date in iOS 15 and macOS 12 | This Dev Brain by Michal Tynior
Learn how to format a Date using the new APIs introduced in iOS 15 and macOS 12.
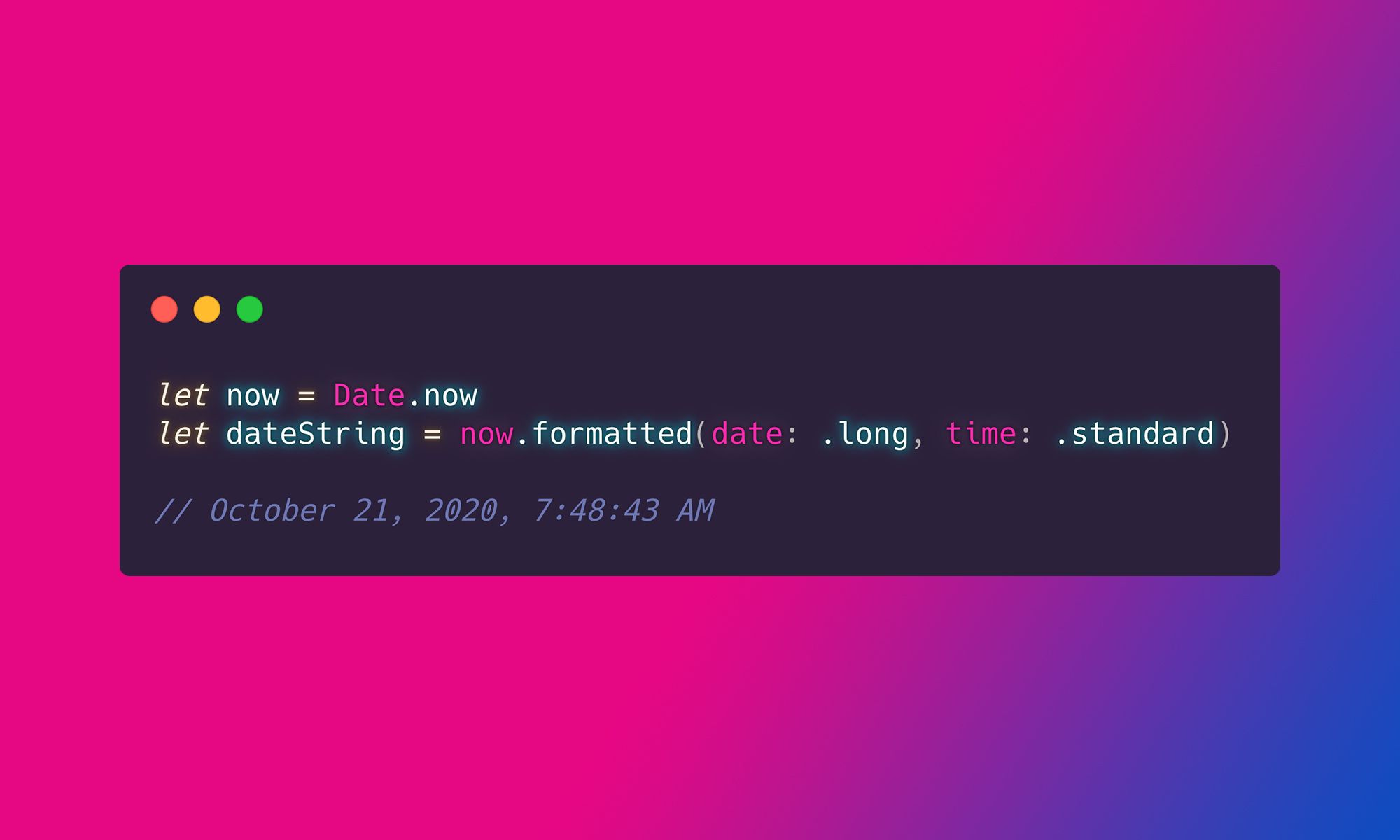
Comments
Anything interesting to share? Write a comment.