Recently I added the Disqus commenting system to this blog. The entire process was super easy. Once I set it up, I started testing everything, and I found an issue I did not anticipate. When I switched the theme, Disqus was not updating itself:
At first, I thought I missed something during the configuration, so I went to the admin panel. After a quick inspection, I was more confused because I set up everything correctly:
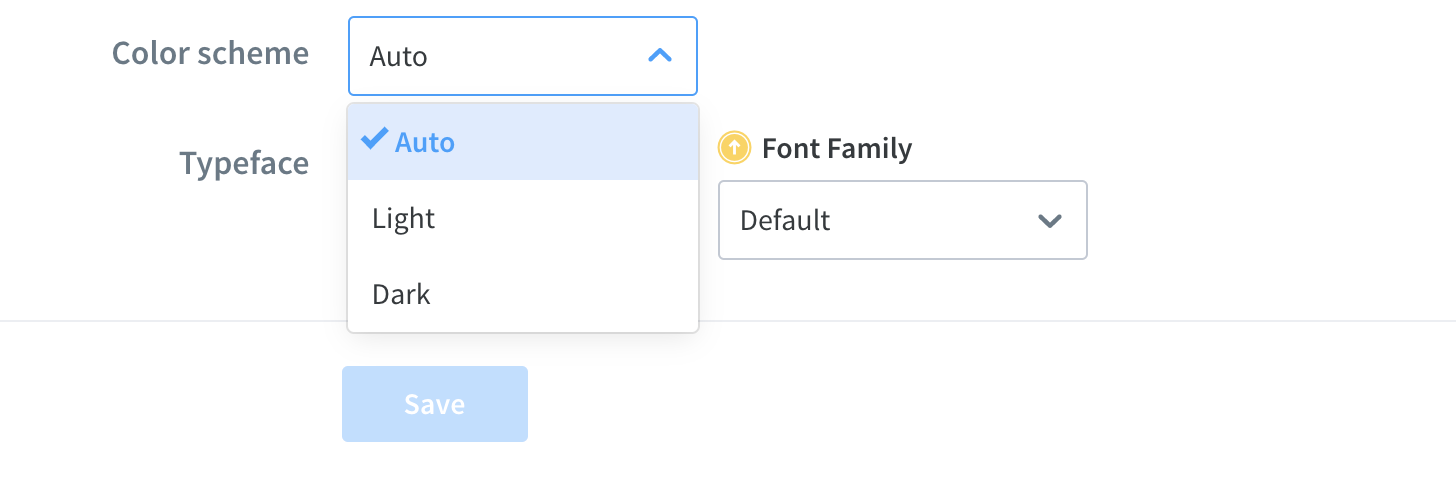
So, I tried one last thing and just reloaded the page, and it worked.
How does the Disqus theme switching work?
It turns out, Disqus sets the color scheme during the initial load. When the auto option is selected, Disqus resolves the theme based on the text color used on the website. If the gray contrast is less than 50%, Disqus will load a dark color scheme. Otherwise, it will use a light theme.
At this point, I knew that to fix this issue I had to either reload the entire page or just the commenting system. The first solution would be the easiest from a development point of view, but it would affect the user experience. The second idea was ideal for readers, but I was afraid it was not possible. Fortunately, it turned that reloading Disqus is super easy.
Reloading Disqus programmatically
Let's start with the setTheme()
function that is triggered every time a reader clicks the theme switcher button:
function setTheme() {
// Logic that sets the theme on the website. Removed for clarity
}
The details of how it changes the themes are not important right now, but we are going to extend this function:
function setTheme() {
// Logic that sets the theme on the website. Removed for clarity
// Create and send event
const event = new Event('themeChanged');
document.dispatchEvent(event);
}
Whenever the theme is changed, the code above will create a custom event named themeChanged
. Then it will propagate (send) this event throughout the page.
Now, we need to start listening for this event and reload the comments system. The best place to add this will be the standard Disqus installation, which should look more or less like this:
<div id="disqus_thread"></div>
<script>
var disqus_config = function () {
this.page.url = PAGE_URL; // Replace PAGE_URL with your page's canonical URL variable
this.page.identifier = PAGE_IDENTIFIER; // Replace PAGE_IDENTIFIER with your page's unique identifier variable
};
(function() {
var d = document, s = d.createElement('script');
s.src = 'https://SITE_URL.disqus.com/embed.js'; // Replace SITE_URL with your site's URL
s.setAttribute('data-timestamp', +new Date());
(d.head || d.body).appendChild(s);
})();
</script>
<noscript>Please enable JavaScript to view the <a href="https://disqus.com/?ref_noscript">comments powered by Disqus.</a></noscript>
We can add the code that reloads Disqus every time it receives the themeChanged
event, just right before the </script>
tag:
<div id="disqus_thread"></div>
<script>
// Disqus configuration. Removed for clarity
// Disqus theme switching
document.addEventListener('themeChanged', function (e) {
if (document.readyState == 'complete') {
DISQUS.reset({ reload: true, config: disqus_config });
}
});
</script>
<noscript>Please enable JavaScript to view the <a href="https://disqus.com/?ref_noscript">comments powered by Disqus.</a></noscript>
The code above adds, to the page, an event listener that listens for the themeChanged
. Once it receives this event, it checks whether the page is already loaded. We don't need to reload the comments when a page is loading because, at this point, Disqus will set the proper color scheme. When the page is already loaded, we need to force Disqus to refresh its state, and we do it by calling Disqus.reset()
function. This function can load or reload comments for a specific thread. That's why it requires information about the disqus_config
.
That's it. Now, when we change the theme, Disqus applies the proper color scheme:
It wasn't too hard, was it? With a couple of lines of javascript, we have automatic Disqus theme switching.
I hope this was helpful, and you know how to do it on your website.
Comments
Anything interesting to share? Write a comment.